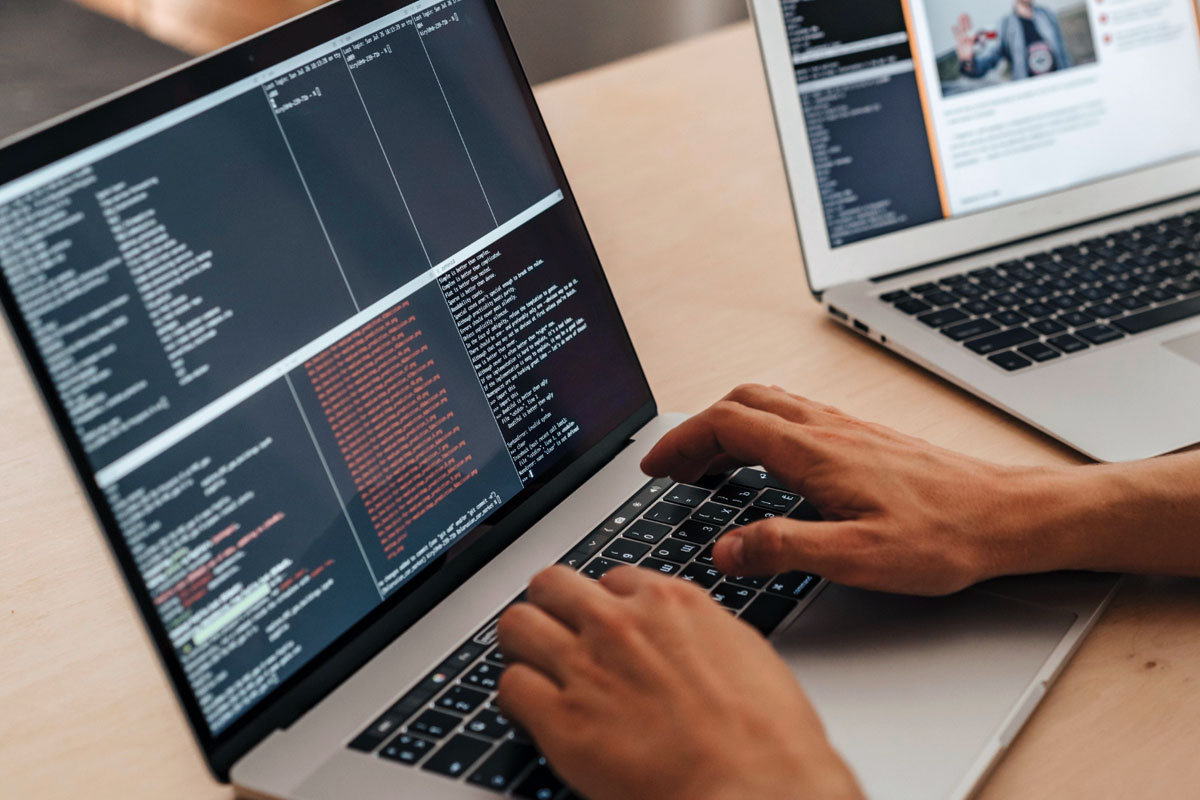
The Post/Redirect/Get (PRG) design pattern is a powerful tool for managing form submissions in web applications. This article will provide a step-by-step guide to implementing PRG, complete with code examples and best practices for effective use.
Step-by-Step Guide to Implementation
Step 1: Create the Form
First, create a form that users can fill out and submit. This form will send data to the server using the POST method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Form</title>
</head>
<body>
<form action="/submit-form" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<br>
<button type="submit">Submit</button>
</form>
</body>
</html>
Handle the POST Request
Next, handle the form submission on the server side. This involves processing the form data and then redirecting the user to a new page.
Here is an example using Node.js with Express:
const express = require('express');
const app = express();
const bodyParser = require('body-parser');
app.use(bodyParser.urlencoded({ extended: true }));
app.post('/submit-form', (req, res) => {
const { name, email } = req.body;
// Process the form data (e.g., save to database)
console.log(`Name: ${name}, Email: ${email}`);
// Redirect to the thank you page
res.redirect('/thank-you');
});
app.get('/thank-you', (req, res) => {
res.send('<h1>Thank you for submitting the form!</h1>');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, the server processes the form data and then responds with a redirect to the /thank-you
page.
Create the Redirect Page
Create the page that users will be redirected to after submitting the form. This can be a simple “Thank You” page or a more detailed confirmation page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Thank You</title>
</head>
<body>
<h1>Thank you for submitting the form!</h1>
<p>We have received your information and will get back to you shortly.</p>
</body>
</html>
Best Practices for Effective Use
- Clear User Feedback: Ensure that users receive clear feedback after submitting a form. The redirect page should confirm that their submission was successful and provide any necessary follow-up information.
- Handle Validation Errors: If there are validation errors during form submission, redirect the user back to the form with appropriate error messages. This can be done by storing errors in the session and displaying them on the form page.
- Avoid Data Loss: Ensure that user input is not lost if there are validation errors or other issues during submission. You can achieve this by repopulating the form with the user’s input upon redirect.
- Security Considerations: Always validate and sanitize user input on the server side to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS).
- SEO Considerations: Use 301 redirects (permanent) for PRG to ensure that search engines index the correct pages. This helps in maintaining SEO value and preventing duplicate content issues.
- Session Management: If using sessions to store data between the POST and GET requests, ensure that session management is handled securely and efficiently.
Handling Validation Errors
Here’s how you might handle validation errors in an Express.js application:
app.post('/submit-form', (req, res) => {
const { name, email } = req.body;
let errors = [];
if (!name) {
errors.push('Name is required');
}
if (!email) {
errors.push('Email is required');
}
if (errors.length > 0) {
req.session.errors = errors;
req.session.success = false;
res.redirect('/');
} else {
// Process the form data (e.g., save to database)
console.log(`Name: ${name}, Email: ${email}`);
req.session.success = true;
res.redirect('/thank-you');
}
});
app.get('/', (req, res) => {
res.render('form', {
errors: req.session.errors,
success: req.session.success
});
req.session.errors = null;
});
In this example, validation errors are stored in the session and displayed on the form page. This ensures that users are informed about any issues with their submission and can correct them without losing their input.
Implementing the Post/Redirect/Get design pattern in web applications is a straightforward yet powerful way to improve user experience and ensure data integrity. By following the step-by-step guide and best practices outlined above, you can effectively implement PRG in your web projects. The next article in this series will explore the advantages of using the PRG design pattern, highlighting how it enhances user experience, prevents duplicate submissions, and improves application reliability.