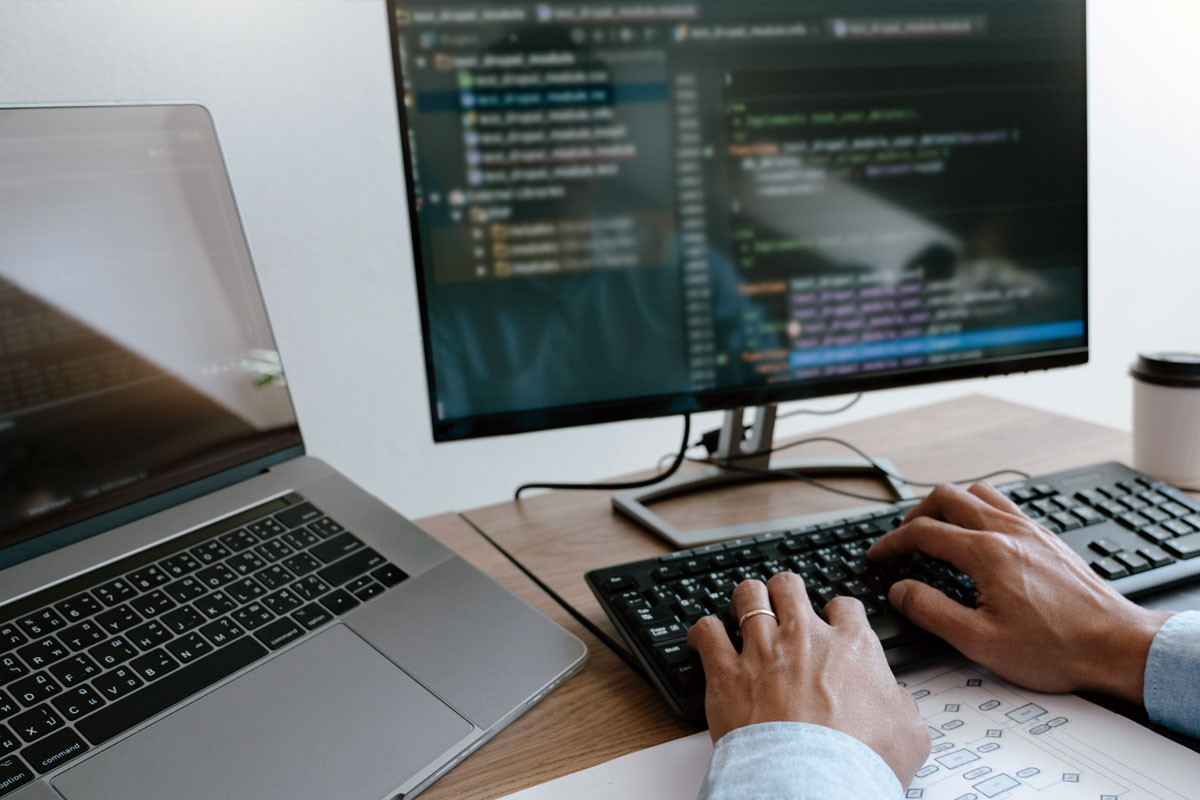
Implementing the Post/Redirect/Get (PRG) design pattern in web applications can greatly enhance user experience and data integrity. However, like any development pattern, it can come with its own set of challenges. This article will cover common challenges and solutions, provide debugging tips, and suggest useful tools and resources to help you successfully implement and troubleshoot PRG in your projects.
Common Challenges and Solutions
Challenge 1: Misconfigured Redirects
Problem: Incorrectly set up redirects can lead to broken workflows where the user does not get redirected properly after form submission, leading to confusion and potential data loss.
Solution:
- Verify URLs: Ensure that the redirect URLs are correctly specified and that they point to valid and accessible endpoints.
- Status Codes: Use appropriate HTTP status codes (typically 302 for temporary redirects or 303 for see-other redirects).
- Server Logs: Check server logs to identify any issues with redirect responses.
Example:
// Express.js example
app.post('/submit-form', (req, res) => {
const { name, email } = req.body;
if (name && email) {
// Process data
res.redirect(303, '/thank-you'); // Correctly using 303 status code
} else {
res.redirect(303, '/form-error'); // Redirect to error page if data is missing
}
});
Challenge 2: Session Management Issues
Problem: Managing session data between the POST and GET requests can be tricky. If not handled correctly, session data might be lost or not properly utilized.
Solution:
- Consistent Session Handling: Ensure that session data is consistently stored and retrieved across different routes.
- Session Expiry: Check that session expiry settings are appropriate for your use case to avoid premature data loss.
Example:
// Express.js with session example
const session = require('express-session');
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true,
cookie: { maxAge: 60000 } // Set appropriate session expiry time
}));
app.post('/submit-form', (req, res) => {
req.session.formData = req.body;
res.redirect(303, '/thank-you');
});
app.get('/thank-you', (req, res) => {
if (req.session.formData) {
// Process session data
res.send('<h1>Thank you for submitting the form!</h1>');
} else {
res.redirect('/'); // Redirect if no session data found
}
});
Challenge 3: Handling Validation Errors
Problem: If validation errors occur during form submission, it can be challenging to provide users with meaningful feedback while preserving their input data.
Solution:
- Store Errors in Session: Store validation errors and user input in the session and retrieve them upon redirect.
- Display Errors: Display error messages clearly on the form page, allowing users to correct their input without re-entering all data.
Example:
app.post('/submit-form', (req, res) => {
const { name, email } = req.body;
let errors = [];
if (!name) errors.push('Name is required');
if (!email) errors.push('Email is required');
if (errors.length > 0) {
req.session.errors = errors;
req.session.formData = req.body;
res.redirect('/form');
} else {
// Process data
res.redirect(303, '/thank-you');
}
});
app.get('/form', (req, res) => {
const errors = req.session.errors || [];
const formData = req.session.formData || {};
req.session.errors = null;
req.session.formData = null;
res.render('form', { errors, formData });
});
Debugging Tips
- Use Browser Developer Tools: Use the network tab in browser developer tools to monitor HTTP requests and responses. Check for correct status codes and redirect URLs.
- Log Everything: Add logging statements to your server-side code to track the flow of requests and identify where issues occur.
- Check Session Data: Inspect session data at different points to ensure it is being correctly stored and retrieved.
- Test Redirects: Manually test all form submission paths, including both successful submissions and submissions with validation errors, to ensure redirects are functioning as expected.
Useful Tools and Resources
- Postman: Use Postman to manually test your API endpoints and ensure they are handling POST and GET requests correctly.
- Browser Developer Tools: Utilize tools like Chrome DevTools or Firefox Developer Tools to inspect network requests, session data, and console logs.
- Express.js Documentation: Refer to the official Express.js documentation for detailed information on handling requests, responses, and sessions.
- OWASP Guidelines: Follow the OWASP guidelines for secure coding practices, particularly for handling form data and session management.
Implementing the PRG design pattern can significantly improve the reliability and user experience of your web applications. By understanding and addressing common challenges, utilizing effective debugging techniques, and leveraging useful tools, you can successfully implement and troubleshoot PRG in your projects. This concludes our series on the PRG design pattern. By following the practices discussed across these articles, you can build robust and user-friendly web applications that effectively handle form submissions.